Chat Widget V1
- Getting Started
- Chat Widget Methods
- Chat Widget Events
- Chat Widget Snipped Code Configuration
- Content Security Policy
- CSP Domain Description
- Chat Widget Scenario Best Practices Use Cases
Getting Started
Chat Widget is a JSSDK (hereafter referred to as Chat widget) that allows embedding into your own website, it comes in handy whenever contacts want to contact you, more detailed configuration and usage documents are here. This document mainly focuses on JSSDK related content.
Chat Widget Methods
Open chat widget
webchat.selfHostedApp.open()
Close chat widget
webchat.selfHostedApp.close()
Set context
The following information should be taken into account when setting the context of the chat widget:
- Does not support complex schema, it only currently supports the key value and the value type, which can only be a string or number.
- It is only passed to Talkdesk Studio when a conversation is first created.
- Calling this method to update an ongoing conversation cannot be synchronized to the conversation app context card.
webchat.setContextParam({ "var1": "value1", "var2": "value2", "var3": 100 })
Set the default value of Initial Screen form
When you enable the Initial Screen function in Admin, if you need to add some default values to the form, the field name passed in can be seen in the admin chat touchpoint
webchat.setCustomFieldDefaultValues({ "field_email1": "[[email protected]](mailto:[email protected])","field_number1": "+1" })
Chat Widget Events
Initialization completed
webchat.init(configs).then(function() {
// Some things you want to do after Chat JSSDK is initialized
})
When chat is opened
webchat.onOpen = function () {
// Some things you want to do after chat is opened
}
When chat is closed
webchat.onClose = function () {
// Some things you want to do after chat is closed
}
When the conversation starts
webchat.onConversationStart = function () {
// Some things you want to do after the conversation start
}
Chat Widget Snippet Code Configuration
Field name | Type | Is required | Description |
---|---|---|---|
window | The browser's built-in window reading item | Yes | Browser built-in window object |
document | The browser's built-in document reading item | Yes | Browser built-in document object |
node | String | Yes | The target dom node ID to which chat ui is mounted (currently, this parameter value must be tdWebchat) |
props | See props configuration below for details | Yes | Mainly responsible for controlling function switches |
configs | See configs configuration below for details | Yes | Mainly responsible for setting chat style |
props configuration
Field name | Type | Is required | Default value | Description |
---|---|---|---|---|
touchpointId | string | Yes | Chat touchpoint unique identification ID, used to obtain chat jssdk configuration | |
accountId | string | No | For historical reasons, please set it to null or empty string by default. | |
region | enum | Yes | The area of the Talkdesk endpoints gateway used by chat. The currently supported enumeration values are: td-us-1 td-eu-1 td-ca-1 td-usfed-1 | |
languageCode | enum | No | en-US | Internationalized language for chat initialization The current supported enumeration values are: en-US pt-PT es-ES de-DE fr-FR it-IT pt-BR |
configs configuration
Field name | Type | Is required | Default value | Description |
---|---|---|---|---|
enableEmoji | boolean | No | True | Control for emoji, hidden or displayed. |
enableValidation | boolean | No | False | Control for validate for initial screen from the email or phone number input. |
enableUserInput | boolean | No | True | Control for Send button and input, hidden or displayed. |
enableAttachments | boolean | True | en-US | Control for attachment button, hidden or displayed. |
enableResponsiveLayout | boolean | No | True | Control whether the automatic full-screen capability is enabled. |
languageCode | enmus | No | en-US | Control which language the chat uses, higher than props param settings. The currently supported enumeration values are: en-US pt-PT es-ES de-DE fr-FR it-IT pt-BR |
styles | object | No | See configs.styles configuration below for details | |
configs.styles configuration
Customizing the widget launch:
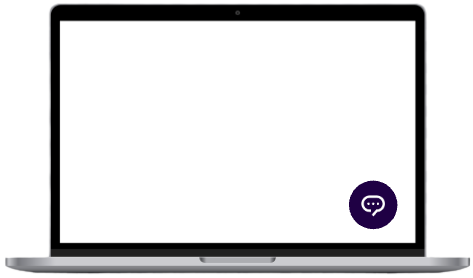
Field name | Type | Default value | Description |
---|---|---|---|
chatThemeColor | string | # 1E0044 | Color of the launcher background and the header. |
chatHoverThemeColor | string | # 1A223F | Color of the launcher background and the header. |
chatIcon | string | https://qa-cdn-talkdesk.talkdeskdev.com/cdn-assets/latest/talkdesk/product/app-icons/sms.svg | The icon of the launcher. Note: Best format: SVG, although it supports other formats such as PNG and JPG. For a round shape, it should have a transparent background. |
triggerButtonWidth | string | 35px | Width of the icon of the launcher. Note: It won’t exceed the launcher’s size; therefore, its maximum value is 64 px. |
triggerButtonHeight | string | 35px | Height of the icon of the launcher. Note: It won’t exceed the launcher’s size; therefore, its maximum value is 64 px. |
triggerButtonPositionRight | string | 30px | Distance of the launcher to the right side of the window. |
triggerButtonPositionBottom | string | 40px | Distance of the launcher to the bottom of the window. |
Customizing the widget window:
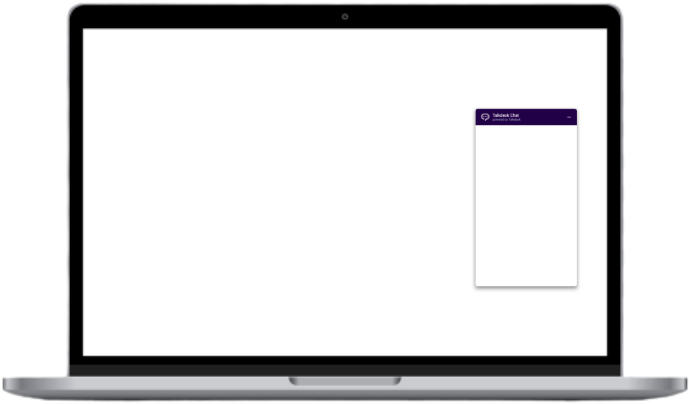
Field name | Default value | Type | Description |
---|---|---|---|
chatContainerWidth | string | 335px | Width when Chat is maximized |
chatContainerHeight | string | 600px | Height when Chat is maximized |
chatPositionBottom | string | 20px | The distance from the bottom of the screen when Chat is maximized |
chatThemeMainFont | string | Roboto-Regular, sans-serif | Font of the subtitle, input box placeholder, chat messages, and notifications. Note: It supports web-safe fonts. If you wish to use a non-supported font, we recommend pre-installing the font on your website. |
Customizing the widget header:

Field name | Default value | Type | Description |
---|---|---|---|
chatTitleIcon | string | 32px | The icon of the header left. |
chatTitleIconWidth | string | 32px | Width of the icon of the header left. |
chatTitleIconHeight | string | # 1E0044 | Height of the icon of the header left. |
chatThemeColor | string | Talkdesk Chat | Color of the launcher background and the header. |
chatTitle | string | Title of the header. | |
chatTitleTextColor | string | Powered by Talkdesk | Font color of the title of the header. |
chatCloseButtonColor | string | # ffffff | Color of the close chat conversation button. |
chatMinimizeButtonColor | string | # ffffff | Color of the minimize widget button. |
Customizing the initial screen:
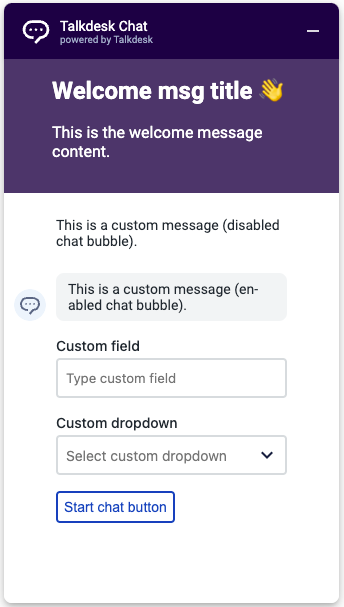
Field name | Type | Default value | Description |
---|---|---|---|
welcomeMessageBackgroundColor | string | # 4D356A | Background color of the welcome message. |
welcomeMessageTitleFontFamily | string | Roboto-Regular, sans-serif | Font of the title of the welcome message. |
welcomeMessageTitleFontSize | string | 24px | Font size of the title of the welcome message. |
welcomeMessageTitleColor | string | # ffffff | Font color of the title of the welcome message. |
welcomeMessageContentFontFamily | string | Roboto-Regular, sans-serif | Font of the content of the welcome message. |
welcomeMessageContentFontSize | string | 16x | Font size of the content of the welcome message. |
welcomeMessageContentColor | string | # ffffff | Font color of the content of the welcome message. |
customMessageNoBubbleFontFamily | string | Roboto-Regular, sans-serif | Font of the custom message with no bubble. |
customMessageNoBubbleFontSize | string | 14px | Font size of the custom message with no bubble. |
customMessageNoBubbleColor | string | # 202830 | Font color of the custom message with no bubble. |
customMessageBackgroundColor | string | # F2F4F5 | Background color of the custom message with bubble. |
customMessageFontFamily | string | Roboto-Regular, sans-serif | Font of the custom message with bubble. |
customMessageFontSize | string | 14px | Font size of the custom message with bubble. |
customMessageColor | string | # 202830 | Font color of the custom message with bubble. |
customFieldLabelFontFamily | string | Roboto-Regular, sans-serif | Font of the label of a custom field. |
customFieldLabelFontSize | string | 14px | Font size of the label of a custom field. |
customFieldLabelColor | string | # 202830 | Font color of the label of a custom field. |
customFieldInputFontFamily | string | Roboto-Regular, sans-serif | Font of the input text of a custom field. |
customFieldInputFontSize | string | 14px | Font size of the input text of a custom field. |
customFieldInputColor | string | # 202830 | Font color of the input text of a custom field. |
customFieldInputBackgroundColor | string | # ffffff | Background color of a custom field. |
customFieldInputPlaceholder | string | Type | Partial placeholder of a custom field. |
customDropdownLabelFontFamily | string | Roboto-Regular, sans-serif | Font of the label of a custom dropdown. |
customDropdownLabelFontSize | string | 14px | Font size of the label of a custom dropdown. |
customDropdownLabelColor | string | # 202830 | Font color of the label of a custom dropdown. |
customDropdownInputFontFamily | string | Roboto-Regular, sans-serif | Font of the input text of a custom dropdown. |
customDropdownInputFontSize | string | 14px | Font size of the input text of a custom dropdown. |
customDropdownInputColor | string | # 202830 | Font color of the input text of a custom dropdown. |
customDropdownInputBackgroundColor | string | # ffffff | Background color of a custom dropdown. |
customDropdownInputPlaceholder | string | Select | Partial placeholder of a custom dropdown. |
customButtonFontFamily | string | Roboto-Regular, sans-serif | Font of the label of a custom button. |
customButtonFontSize | string | 14px | Font size of the label of a custom button. |
customButtonColor | string | # 003FBD | Font size of the label of a custom button. |
customButtonBackgroundColor | string | # ffffff | Background color of a custom button. |
customButtonColor | string | # 003FBD | Font color of the label of a custom button |
customButtonBackgroundColor | string | # ffffff | Background color of a custom button. |
customButtonBorderColor | string | # 003FBD | Border color of a custom button. |
customButtonHeight | string | 32px | Height of a custom button. |
customFieldPhoneDescription | string | Include the country code, avoid spaces and/or symbols (e.g. +1123456789) | Phone number input Prompt user description Note: This item will only be displayed if configs.enableValidation is set to true. see 4.6 for details. |
customFieldPhoneInvalidFormatMessage | string | Please type a valid phone number | Prompt message when phone number input fails verification Note: This item will only be displayed if configs.enableValidation is set to true. see 4.6 for details. |
customFieldEmailInvalidFormatMessage | string | 14px | Prompt message when email input fails verification.Prompt message when phone number input fails verification Note: This item will only be displayed if configs.enableValidation is set to true. see 4.6 for details. Note: This item will only be displayed if configs.enableValidation is set to true. see 4.6 for details. |
Customizing the conversation
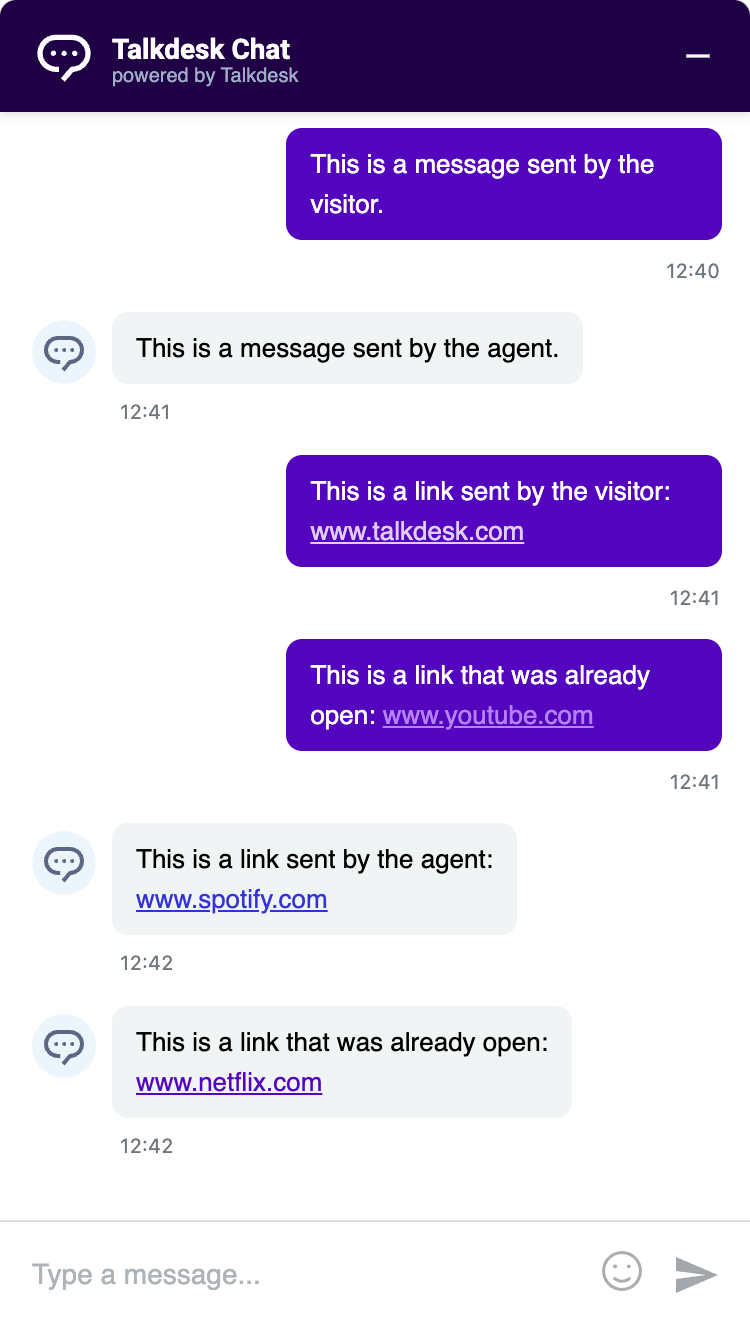
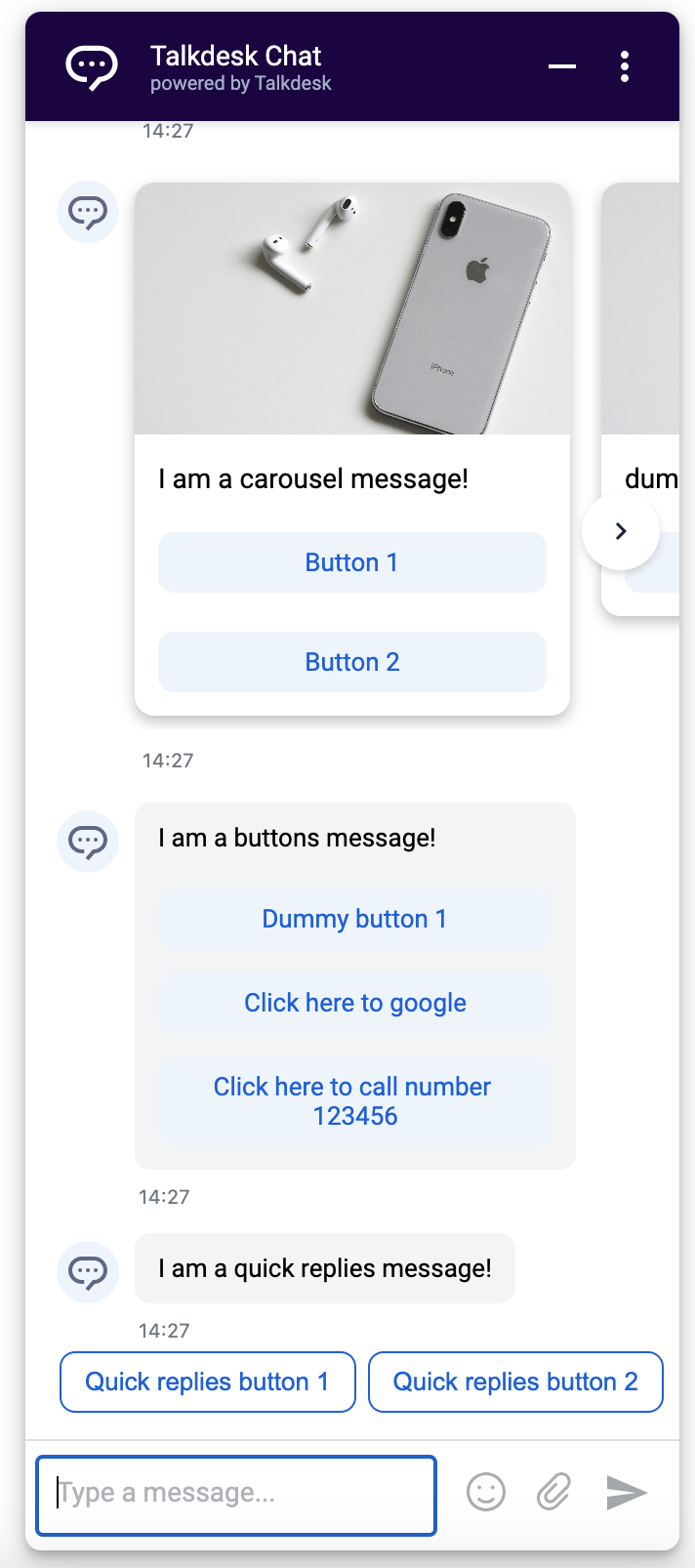
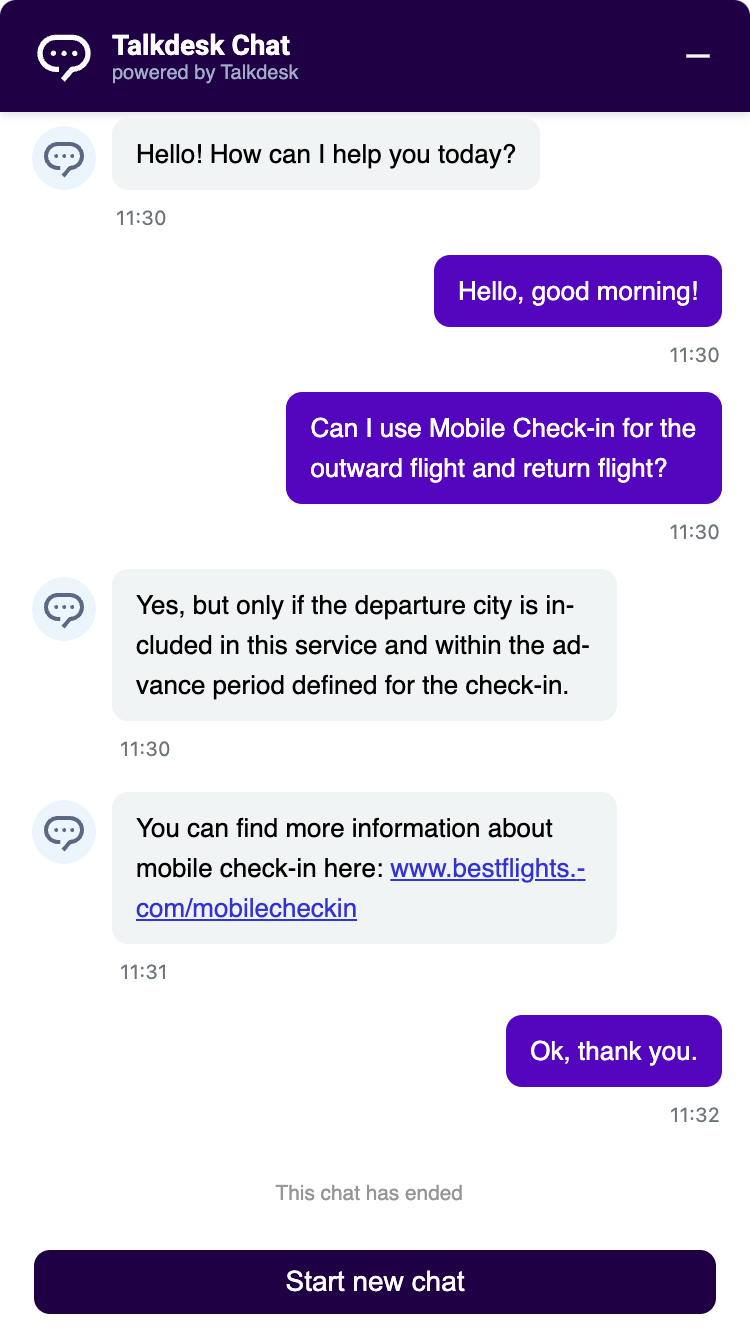
Field name | Type | Default value | Description |
---|---|---|---|
userTextColor | string | # ffffff | Font color of a message sent by a visitor. |
userBackgroundColor | string | # 5405BD | Background color of a message sent by a visitor. |
botTextColor | string | # 00000 | Agent or VA agent message bubble font color. |
botBackgroundColor | string | # F2F4F5 | Background color of a message sent by an agent or a bot. |
userAnchorTextColor | string | # E1CCFE | Font color of an unvisited hyperlink sent by a visitor. |
userAnchorTextColorVisited | string | # B681FC | # 5405BDFont color of a visited hyperlink sent by an agent or a bot. |
botAnchorTextColor | string | # 3232D6 | # 3232D6Font color of an unvisited hyperlink sent by an agent or a bot. |
botAnchorTextColorVisited | string | # 5405BD | # 5405BDFont color of a visited hyperlink sent by an agent or a bot. |
botIconVerticalAlign | string | top | Placement of the agent avatar towards the message bubble. It can be ‘top’ or ‘bottom’. |
avatarForegroundColor | string | # 5C6784 | Foreground color of the default agent avatar. |
avatarBackgroundColor | string | # EDF4FC | Background color of the default agent avatar. |
avatarBorderColor | string | # EDF4FC | Border color of the default agent avatar. |
botIconHeight | string | 32px | Height of the agent avatar. |
botIconWidth | string | 32px | Width of the agent avatar. |
botIcon | string | Custom agent avatar. Note: It overrides all the default agent avatar properties. | |
userInputSeparatorColor | string | # DFDFDF | Color of the separator above the input box. |
userInputHeight | string | 56px | Minimum height of user message input box. |
chatPlaceholder | string | Type a message... | Placeholder of the input box. |
buttonTextColor | string | # 005cde | Set the font color of all VA Buttons and buttons in the carousel component. |
buttonHoverTextColor | # 005cde | Set the font color of all VA Buttons and buttons in the carousel component when the mouse is moved into it. | |
buttonBackgroundColor | string | rgb(237, 244, 252) | Set the background color of all VA Buttons and buttons in the carousel component. |
buttonHoverBackgroundColor | string | rgb(237, 244, 252) | Set the background color of all VA Buttons and buttons in the carousel component when the mouse is moved into it. |
replyTextColor | string | # 005cde | Set the font color of all VA quick replay buttons. |
replyHoverTextColor | string | # edf4fc | Set the font color of all VA quick replay buttons when the mouse is moved into them. |
replyBorderColor | string | # 005cde | Set the border color of all VA quick replay buttons. |
replyBackgroundColor | string | transparent | Set the background color of all VA quick replay buttons. |
endedChatMessage | string | End chat | System message text displayed at the end of the conversation. |
startChatButtonTextColor | string | # ffffff | Start new conversation button font color. |
startChatButtonHoverTextColor | string | # ffffff | The font color of the Start New Conversation button when the mouse is moved into it. |
startChatButtonBorderColor | string | # 1e0044 | Start new conversation button border color. |
startChatButtonHoverBorderColor | string | # 1e0044 | The color of the border when the mouse is moved into the Start New Conversation button. |
startChatButtonBackgroundColor | string | # 1e0044 | Start new conversation button background color |
startChatButtonHoverBackgroundColor | string | # 1e0044 | The background color of the Start New Conversation button when the mouse is moved into it. |
startChatButtonLabel | string | Start new chat | Display copy in Start New Conversation button. |
Customizing the close conversation dialog for contacts
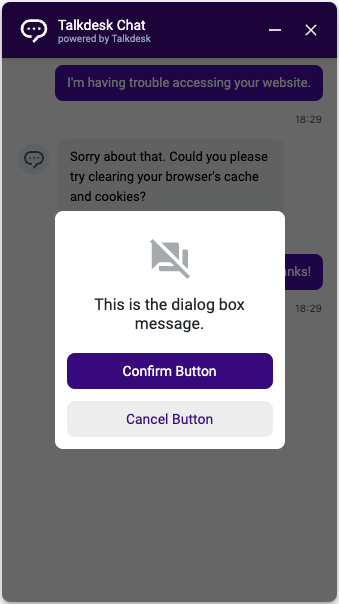
Field name | Type | Default value | Description |
---|---|---|---|
chatDialogBoxIconColor | string | # AEB3B8 | Color of the icon of the dialog box. |
chatDialogBoxFontFamily | string | Roboto-Regular, sans-serif | Font of the message of the dialog box. |
chatDialogBoxFontSize | string | 16px | Font size of the message of the dialog box. |
chatDialogBoxFontColor | string | # 202830 | Font color of the message of the dialog box. |
chatConfirmButtonFontFamily | string | Roboto-Regular, sans-serif | Font of the confirm button of the dialog box. |
chatConfirmButtonFontSize | string | 14px | Font size of the confirm button of the dialog box. |
chatConfirmButtonFontColor | string | # ffffff | Font color of the confirm button of the dialog box. |
chatConfirmButtonBackgroundColor | string | # 3E048B | Background color of the confirm button of the dialog box. |
chatCancelButtonFontFamily | string | Roboto-Regular, sans-serif | Font of the cancel button of the dialog box. |
chatCancelButtonFontSize | string | 14px | Font size of the cancel button of the dialog box. |
chatCancelButtonFontColor | string | # F2F4F5 | Font color of the cancel button of the dialog box. |
chatDialogBoxEndChat | string | Are you sure you want to end this chat? | Message of the dialog box when the contact person tries to end the conversation. |
chatConfirmButtonEndChat | string | Yes, end chat | Label of the confirm button of the dialog box when the contact person tries to end the conversation. |
chatCancelButtonEndChat | string | Cancel | Label of the cancel button of the dialog box when the contact person tries to end the conversation. |
chatDialogBoxRetryEndChat | string | There was an error while ending the chat | Message of the dialog box when an error occurs while attempting to end the conversation. |
chatConfirmButtonRetryEndChat | string | Try again | Label of the confirm button of the dialog box when an error occurs while attempting to end the conversation. |
chatCancelButtonRetryEndChat | string | Dismiss | Label of the cancel button of the dialog box when an error occurs while attempting to end the conversation. |
Content Security Policy (CSP)
When a website has a Content Security Policy (CSP) enabled, it restricts the types of content that can be loaded on the site. To ensure that chat functionalities run properly, specific sources must be whitelisted. Below is a description of the CSP configuration needed for Talkdesk regions, including td-us-1, td-eu-1, td-ca-1, and td-usfed-1, to ensure chat functions properly on the website.
CSP Domain Description
Below is the description of the purpose of CSP domains. Each of these domains plays a crucial role in enabling chat services by specifying trusted sources from which content can be loaded, ensuring both functionality and security for users in their respective regions.
CSP Domains | Description |
---|---|
https://talkdeskchatsdk.talkdeskapp.com | TalkDesk Chat Widget JSSDK remote host |
https://qa-cdn-talkdesk.talkdeskdev.com | TalkDesk Chat Widget JSSDK default remote image host |
https://api.talkdeskapp.com | TalkDesk Gateway US region host |
https://api.talkdeskapp.eu | TalkDesk Gateway EU region host |
https://api.talkdeskappca.com | TalkDesk Gateway CA region host |
https://api.talkdeskapp-pubsec.com | TalkDesk Gateway FedRAMP region host (This is a special area that non-FedRAMP customers do not need to configure) |
https://*.dynatrace.com | TalkDesk Chat Widget JSSDK log reporting gateway, used to record error logs |
wss://tsock.us1.twilio.com | Conversation WebSocket channel in td-us-1 or td-ca-1 |
wss://tsock.ie1.twilio.com | Conversation WebSocket channel in td-eu-1 |
https://mcs.us1.twilio.com | Chat attachment service in td-us-1 or td-ca-1 |
https://mcs.ie1.twilio.com | Chat attachment service in td-eu-1 |
https://media.us1.twilio.com | Chat preview attachment service in td-us-1 or td-ca-1 |
https://media.ie1.twilio.com | Chat preview attachment service in td-eu-1 |
data: | Chat Widget JSSDK sometimes displays local files, so this host is needed |
blob: | Chat Widget JSSDK sometimes displays local files, so this host is needed |
Chat widget scenario best practice cases
Default to Hide Chat Launch Icon, Use Customer's Website Button to Open Chat
When customers need to hide the minimized icon of TD chat and then use a button or link on their website to trigger the maximized TD chat, the following is an example of the integration code.
+<button onclick="webchat && webchat.selfHostedApp.open()">click to show chat</button>
+<button onclick="webchat && webchat.selfHostedApp.close()">click to hide chat</button>
+<a id="show-link">click to show chat</a>
+<a id="hide-link">click to hide chat</a>
+<!--custom js code to trigger td chat-->
+<script>
+ document.getElementById("show-link").onclick = function () {
+ webchat && (webchat.selfHostedApp.open())
+ }
+ document.getElementById("hide-link").onclick = function () {
+ webchat && (webchat.selfHostedApp.close())
+ }
+</script>
<!-- Start of Talkdesk Code -->
<script>
var webchat;
(function(window, document, node, props, configs) {
if (window.TalkdeskChatSDK) {
console.error("TalkdeskChatSDK already included");
return;
}
var divContainer = document.createElement("div");
divContainer.id = node;
document.body.appendChild(divContainer);
var src = "https://talkdeskchatsdk.talkdeskapp.com/talkdeskchatsdk.js";
var script = document.createElement("script");
var firstScriptTag = document.getElementsByTagName("script")[0];
script.type = "text/javascript";
script.charset = "UTF-8";
script.id = "tdwebchatscript";
script.src = src;
script.async = true;
firstScriptTag.parentNode.insertBefore(script, firstScriptTag);
script.onload = function() {
webchat = TalkdeskChatSDK(node, props);
webchat.init(configs);
/*
* Send custom data from your website to TalkDesk!
* If you would like to do it, you need to remove the following commented code and
* modify the webchat.setContextParam parameters to pass in the data you need.
*/
/*function setContext() {
webchat.setContextParam({ "var1": "value1", "var2": "value2", "var3": 100 })
}
// Send data when the chat conversation is initiated
webchat.onConversationStart = function() {
setContext()
}
// Send data when the chat widget is open
webchat.onOpenWebchat = function() {
setContext()
}*/
};
})(
window,
document,
"tdWebchat",
{ touchpointId: "<customer touchpointId>", accountId: "", region: "<customer td region>" },
{
enableValidation: false,
enableEmoji: true,
enableUserInput: true,
enableAttachments: true,
+ styles: {
+ // hidden lanuch chat icon
+ triggerButtonPositionBottom: "-65px",
+ triggerButtonPositionRight: "-65px",
+ },
}
);
</script>
<!-- End of Talkdesk Code -->
Open Chat Widget Automatically on Page Load
The customer needs to automatically open TD chat every time the contact person visits the website. The following is an example of the integration code:
<!-- Start of Talkdesk Code -->
<script>
var webchat;
(function(window, document, node, props, configs) {
if (window.TalkdeskChatSDK) {
console.error("TalkdeskChatSDK already included");
return;
}
var divContainer = document.createElement("div");
divContainer.id = node;
document.body.appendChild(divContainer);
var src = "https://talkdeskchatsdk.talkdeskapp.com/talkdeskchatsdk.js";
var script = document.createElement("script");
var firstScriptTag = document.getElementsByTagName("script")[0];
script.type = "text/javascript";
script.charset = "UTF-8";
script.id = "tdwebchatscript";
script.src = src;
script.async = true;
firstScriptTag.parentNode.insertBefore(script, firstScriptTag);
script.onload = function() {
webchat = TalkdeskChatSDK(node, props);
- webchat.init(configs)
+ webchat.init(configs).then(function() {
+ webchat.selfHostedApp.open()
+ });
/*
* Send custom data from your website to TalkDesk!
* If you would like to do it, you need to remove the following commented code and
* modify the webchat.setContextParam parameters to pass in the data you need.
*/
/*function setContext() {
webchat.setContextParam({ "var1": "value1", "var2": "value2", "var3": 100 })
}
// Send data when the chat conversation is initiated
webchat.onConversationStart = function() {
setContext()
}
// Send data when the chat widget is open
webchat.onOpenWebchat = function() {
setContext()
}*/
};
})(
window,
document,
"tdWebchat",
{ touchpointId: "<customer touchpointId>", accountId: "", region: "<customer td region>" },
{ enableValidation: false, enableEmoji: true, enableUserInput: true, enableAttachments: true }
);
</script>
<!-- End of Talkdesk Code -->
Show or Hide Attachments or Emojis in Chat
If the customer website integrates Chat and needs to turn off attachments or emoticon functions, you can modify these configuration items to turn them on and off.
<!-- Start of Talkdesk Code -->
<script>
var webchat;
(function(window, document, node, props, configs) {
if (window.TalkdeskChatSDK) {
console.error("TalkdeskChatSDK already included");
return;
}
var divContainer = document.createElement("div");
divContainer.id = node;
document.body.appendChild(divContainer);
var src = "https://talkdeskchatsdk.talkdeskapp.com/talkdeskchatsdk.js";
var script = document.createElement("script");
var firstScriptTag = document.getElementsByTagName("script")[0];
script.type = "text/javascript";
script.charset = "UTF-8";
script.id = "tdwebchatscript";
script.src = src;
script.async = true;
firstScriptTag.parentNode.insertBefore(script, firstScriptTag);
script.onload = function() {
webchat = TalkdeskChatSDK(node, props);
webchat.init(configs);
/*
* Send custom data from your website to TalkDesk!
* If you would like to do it, you need to remove the following commented code and
* modify the webchat.setContextParam parameters to pass in the data you need.
*/
/*function setContext() {
webchat.setContextParam({ "var1": "value1", "var2": "value2", "var3": 100 })
}
// Send data when the chat conversation is initiated
webchat.onConversationStart = function() {
setContext()
}
// Send data when the chat widget is open
webchat.onOpenWebchat = function() {
setContext()
}*/
};
})(
window,
document,
"tdWebchat",
{ touchpointId: "<customer touchpointId>", accountId: "", region: "<customer td region>" },
{
enableValidation: false,
+ enableEmoji: true, // if set the value is false then emoji disable
enableUserInput: true,
+ enableAttachments: true // if set the value is false then attachment disable
}
);
</script>
<!-- End of Talkdesk Code -->
Manage Automatic Fullscreen Mode in Chat
If we enable the responsive layout when the screen width is lower than 600px or the height is lower than 620px, the chat widget will be resized to full screen, and if we disable the responsive layout, we’ll not set a full screen for the chat widget at any time.
<!-- Start of Talkdesk Code -->
<script>
var webchat;
(function(window, document, node, props, configs) {
if (window.TalkdeskChatSDK) {
console.error("TalkdeskChatSDK already included");
return;
}
var divContainer = document.createElement("div");
divContainer.id = node;
document.body.appendChild(divContainer);
var src = "https://talkdeskchatsdk.talkdeskapp.com/talkdeskchatsdk.js";
var script = document.createElement("script");
var firstScriptTag = document.getElementsByTagName("script")[0];
script.type = "text/javascript";
script.charset = "UTF-8";
script.id = "tdwebchatscript";
script.src = src;
script.async = true;
firstScriptTag.parentNode.insertBefore(script, firstScriptTag);
script.onload = function() {
webchat = TalkdeskChatSDK(node, props);
webchat.init(configs);
/*
* Send custom data from your website to TalkDesk!
* If you would like to do it, you need to remove the following commented code and
* modify the webchat.setContextParam parameters to pass in the data you need.
*/
/*function setContext() {
webchat.setContextParam({ "var1": "value1", "var2": "value2", "var3": 100 })
}
// Send data when the chat conversation is initiated
webchat.onConversationStart = function() {
setContext()
}
// Send data when the chat widget is open
webchat.onOpenWebchat = function() {
setContext()
}*/
};
})(
window,
document,
"tdWebchat",
{ touchpointId: "<customer touchpointId>", accountId: "", region: "<customer td region>" },
{
enableValidation: false,
enableEmoji: true,
enableUserInput: true,
enableAttachments: true,
+ enableResponsiveLayout: true // if the value is set to false, then responsive layout feature will be disable
}
);
</script>
<!-- End of Talkdesk Code -->
It may not be good to simply disable the full-screen capability. For example, the automatic full-screen capability is still needed on some mobile devices, but you can not use it on a PC. The following is an example.
<!-- Start of Talkdesk Code -->
<script>
var webchat;
(function(window, document, node, props, configs) {
if (window.TalkdeskChatSDK) {
console.error("TalkdeskChatSDK already included");
return;
}
var divContainer = document.createElement("div");
divContainer.id = node;
document.body.appendChild(divContainer);
var src = "https://talkdeskchatsdk.talkdeskapp.com/talkdeskchatsdk.js";
var script = document.createElement("script");
var firstScriptTag = document.getElementsByTagName("script")[0];
script.type = "text/javascript";
script.charset = "UTF-8";
script.id = "tdwebchatscript";
script.src = src;
script.async = true;
firstScriptTag.parentNode.insertBefore(script, firstScriptTag);
script.onload = function() {
webchat = TalkdeskChatSDK(node, props);
webchat.init(configs);
/*
* Send custom data from your website to TalkDesk!
* If you would like to do it, you need to remove the following commented code and
* modify the webchat.setContextParam parameters to pass in the data you need.
*/
/*function setContext() {
webchat.setContextParam({ "var1": "value1", "var2": "value2", "var3": 100 })
}
// Send data when the chat conversation is initiated
webchat.onConversationStart = function() {
setContext()
}
// Send data when the chat widget is open
webchat.onOpenWebchat = function() {
setContext()
}*/
};
})(
window,
document,
"tdWebchat",
{ touchpointId: "<customer touchpointId>", accountId: "", region: "<customer td region>" },
{
enableValidation: false,
enableEmoji: true,
enableUserInput: true,
enableAttachments: true,
+ enableResponsiveLayout: /Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(window.navigator.userAgent),
}
);
</script>
<!-- End of Talkdesk Code -->
Pass Custom Context to TalkDesk Studio when Conversationstart
If the customer website has some values that need to be passed to the conversation app, this can be achieved using the following integrated code in the context card on the right.
Note
If you really need to display it in the Conversations app context card, you also need to set the mapping relationship in the ChatDesk studio context panel associated with Chat.Below is an example of setting a screenshot
Step 1:Click the 'add' button
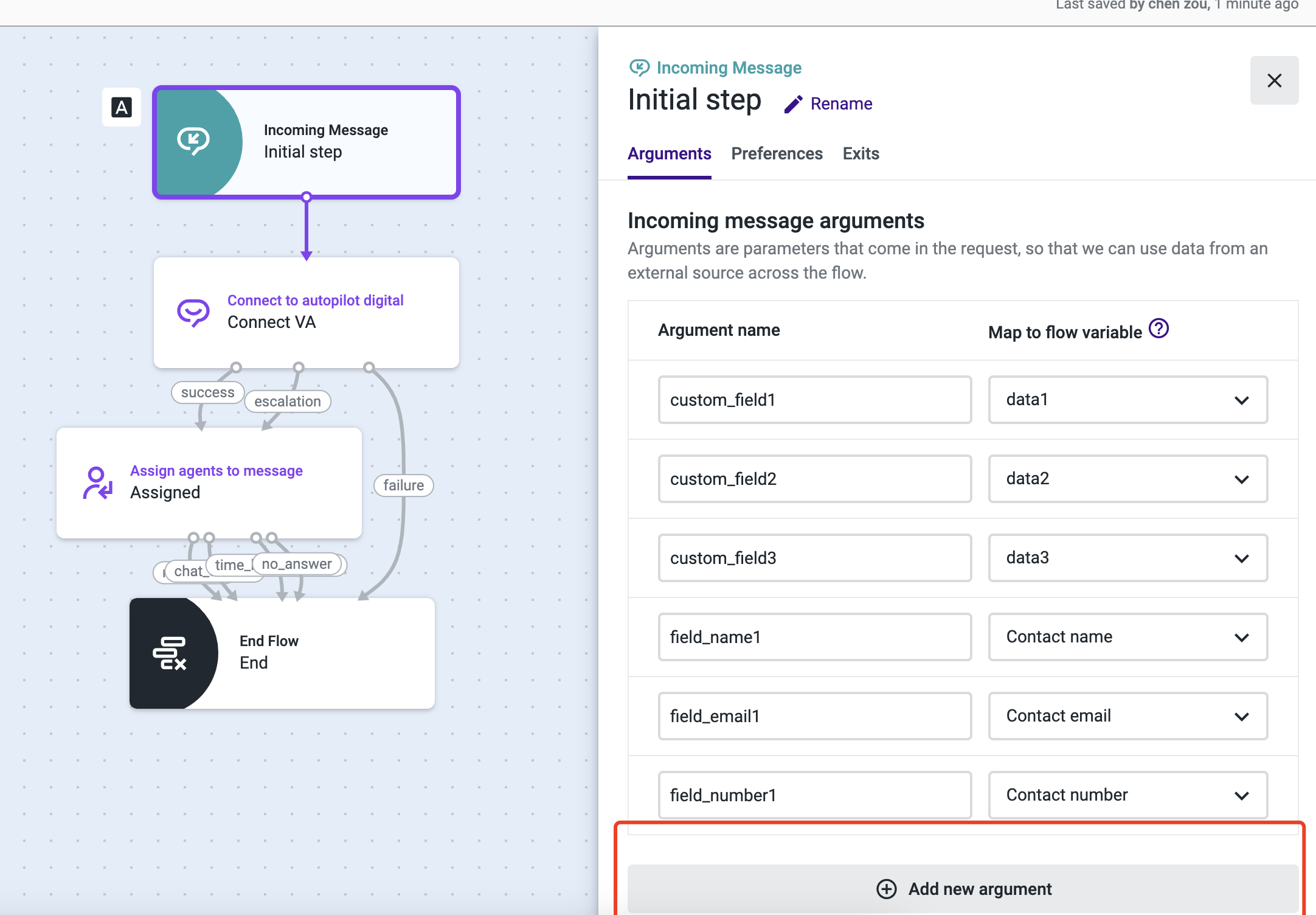
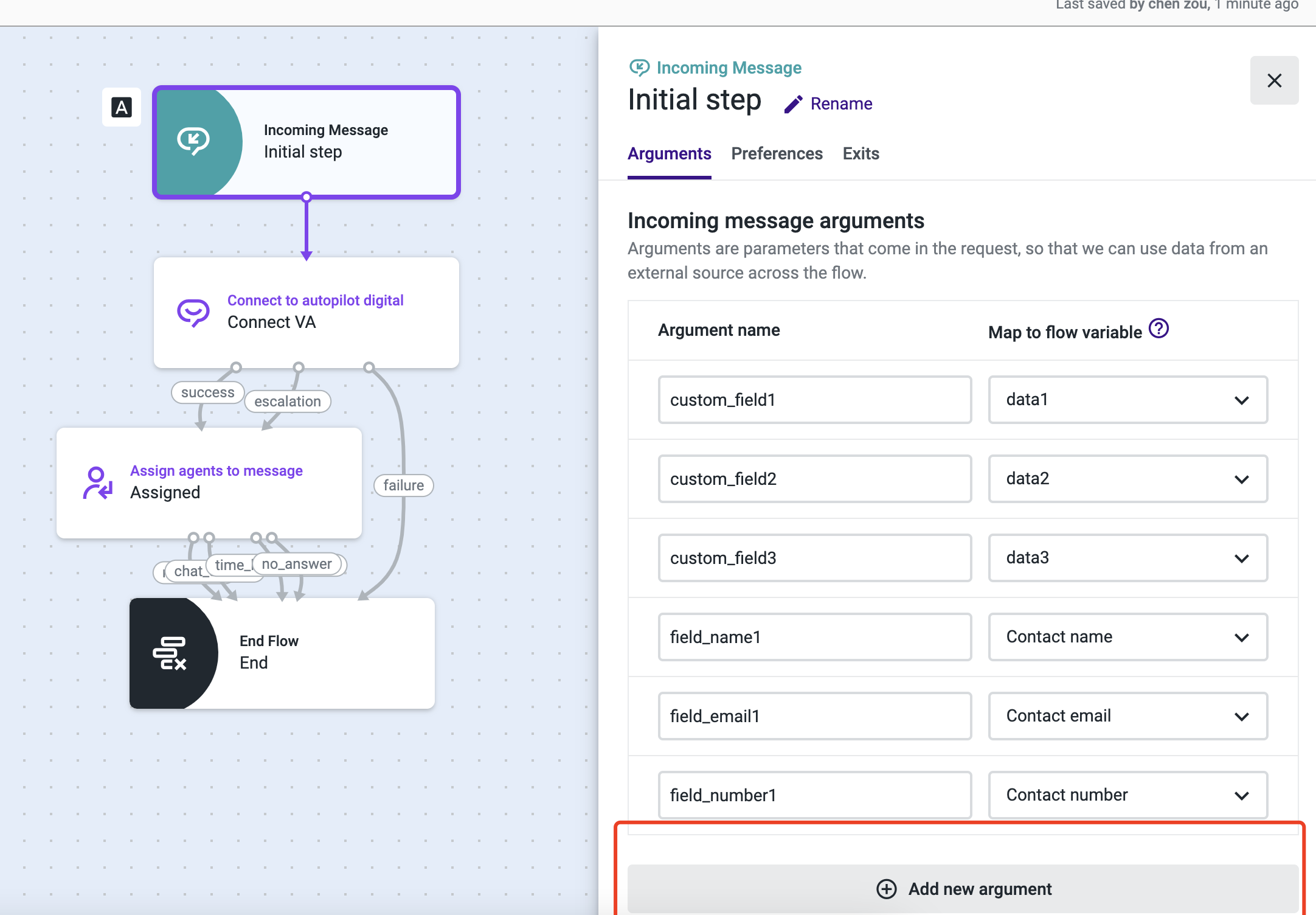
Step 2: Add the context you need to Talkdesk Studio
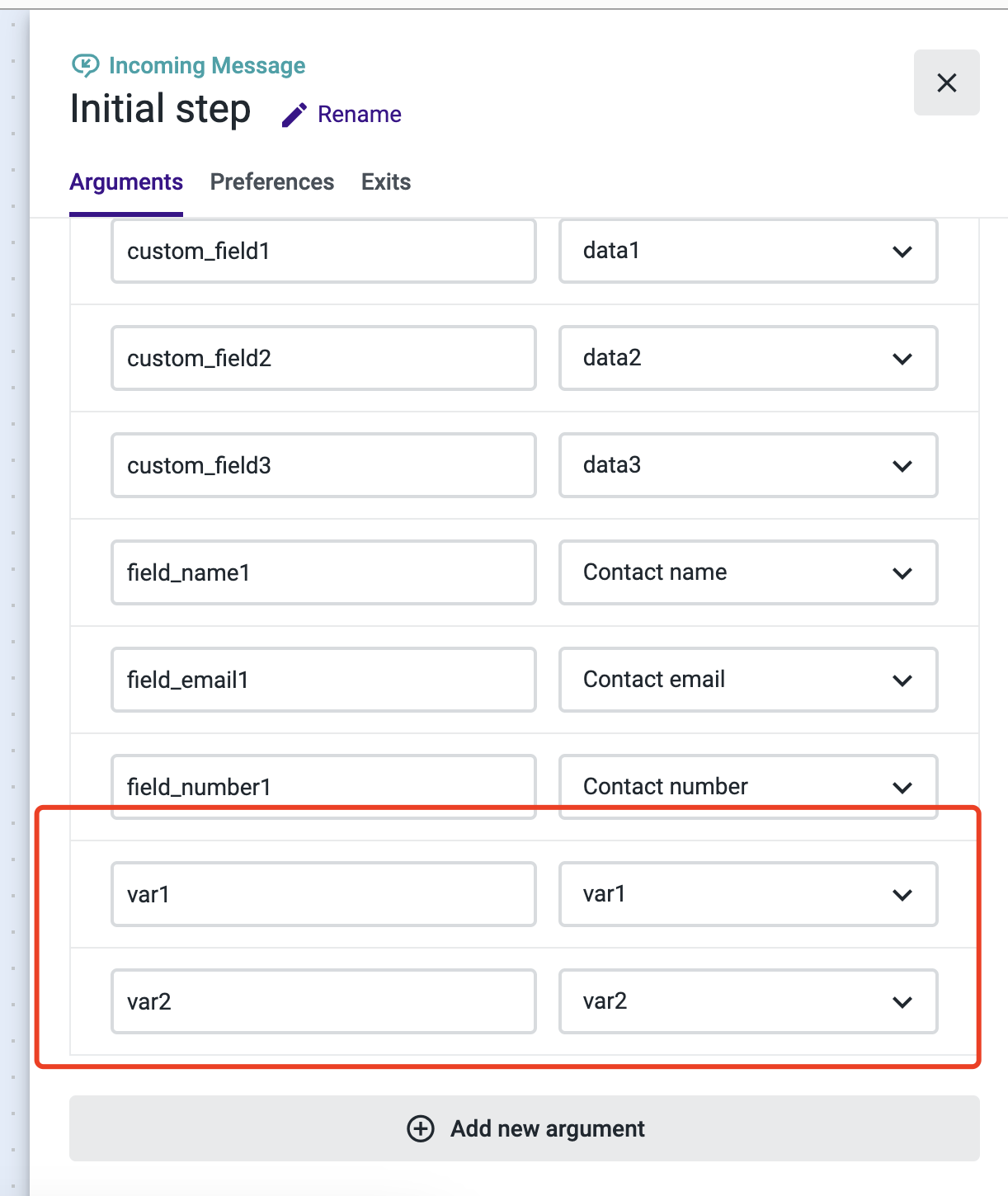
Step 3: Select the context items you need
<!-- Start of Talkdesk Code -->
<script>
var webchat;
(function(window, document, node, props, configs) {
if (window.TalkdeskChatSDK) {
console.error("TalkdeskChatSDK already included");
return;
}
var divContainer = document.createElement("div");
divContainer.id = node;
document.body.appendChild(divContainer);
var src = "https://talkdeskchatsdk.talkdeskapp.com/talkdeskchatsdk.js";
var script = document.createElement("script");
var firstScriptTag = document.getElementsByTagName("script")[0];
script.type = "text/javascript";
script.charset = "UTF-8";
script.id = "tdwebchatscript";
script.src = src;
script.async = true;
firstScriptTag.parentNode.insertBefore(script, firstScriptTag);
script.onload = function() {
webchat = TalkdeskChatSDK(node, props);
webchat.init(configs);
/*
* Send custom data from your website to TalkDesk!
* If you would like to do it, you need to remove the following commented code and
* modify the webchat.setContextParam parameters to pass in the data you need.
*/
- /*function setContext() {
+ function setContext() {
+ // You can modify the object parameters and values in the following methods
webchat.setContextParam({ "var1": "value1", "var2": "value2", "var3": 100 })
}
// Send data when the chat conversation is initiated
webchat.onConversationStart = function() {
setContext()
}
// Send data when the chat widget is open
webchat.onOpenWebchat = function() {
setContext()
- }*/
+ }
};
})(
window,
document,
"tdWebchat",
{ touchpointId: "<customer touchpointId>", accountId: "", region: "<customer td region>" },
{ enableValidation: false, enableEmoji: true, enableUserInput: true, enableAttachments: true }
);
</script>
<!-- End of Talkdesk Code -->
Create and Identify a Contact Automatically
Integrated customers can call the setContextParam method provided by our jssdk on their website to pass the contact person's phone number or email. It must be passed using the td_contact_person_identification field, which currently only supports email or phone number. The following is an example of the integrated code.
<!-- Start of Talkdesk Code -->
<script>
var webchat;
(function(window, document, node, props, configs) {
if (window.TalkdeskChatSDK) {
console.error("TalkdeskChatSDK already included");
return;
}
var divContainer = document.createElement("div");
divContainer.id = node;
document.body.appendChild(divContainer);
var src = "https://talkdeskchatsdk.talkdeskapp.com/talkdeskchatsdk.js";
var script = document.createElement("script");
var firstScriptTag = document.getElementsByTagName("script")[0];
script.type = "text/javascript";
script.charset = "UTF-8";
script.id = "tdwebchatscript";
script.src = src;
script.async = true;
firstScriptTag.parentNode.insertBefore(script, firstScriptTag);
script.onload = function() {
webchat = TalkdeskChatSDK(node, props);
webchat.init(configs);
/*
* Send custom data from your website to TalkDesk!
* If you would like to do it, you need to remove the following commented code and
* modify the webchat.setContextParam parameters to pass in the data you need.
*/
- /*function setContext() {
+ function setContext() {
webchat.setContextParam({
+ // Here, the unique identifier of the contact person is passed to talkdesk. Currently, only phone numbers and email addresses are supported. Please choose one to pass.
+ "td_contact_person_identification": "[email protected]"
})
}
// Send data when the chat conversation is initiated
webchat.onConversationStart = function() {
setContext()
}
// Send data when the chat widget is open
webchat.onOpenWebchat = function() {
setContext()
- }*/
+ }
};
})(
window,
document,
"tdWebchat",
{ touchpointId: "<customer touchpointId>", accountId: "", region: "<customer td region>" },
{ enableValidation: false, enableEmoji: true, enableUserInput: true, enableAttachments: true }
);
</script>
<!-- End of Talkdesk Code -->
Set Default Values for the Initial Screen Form
The field name is generated in the channel's chat touchpoint initial screen form:
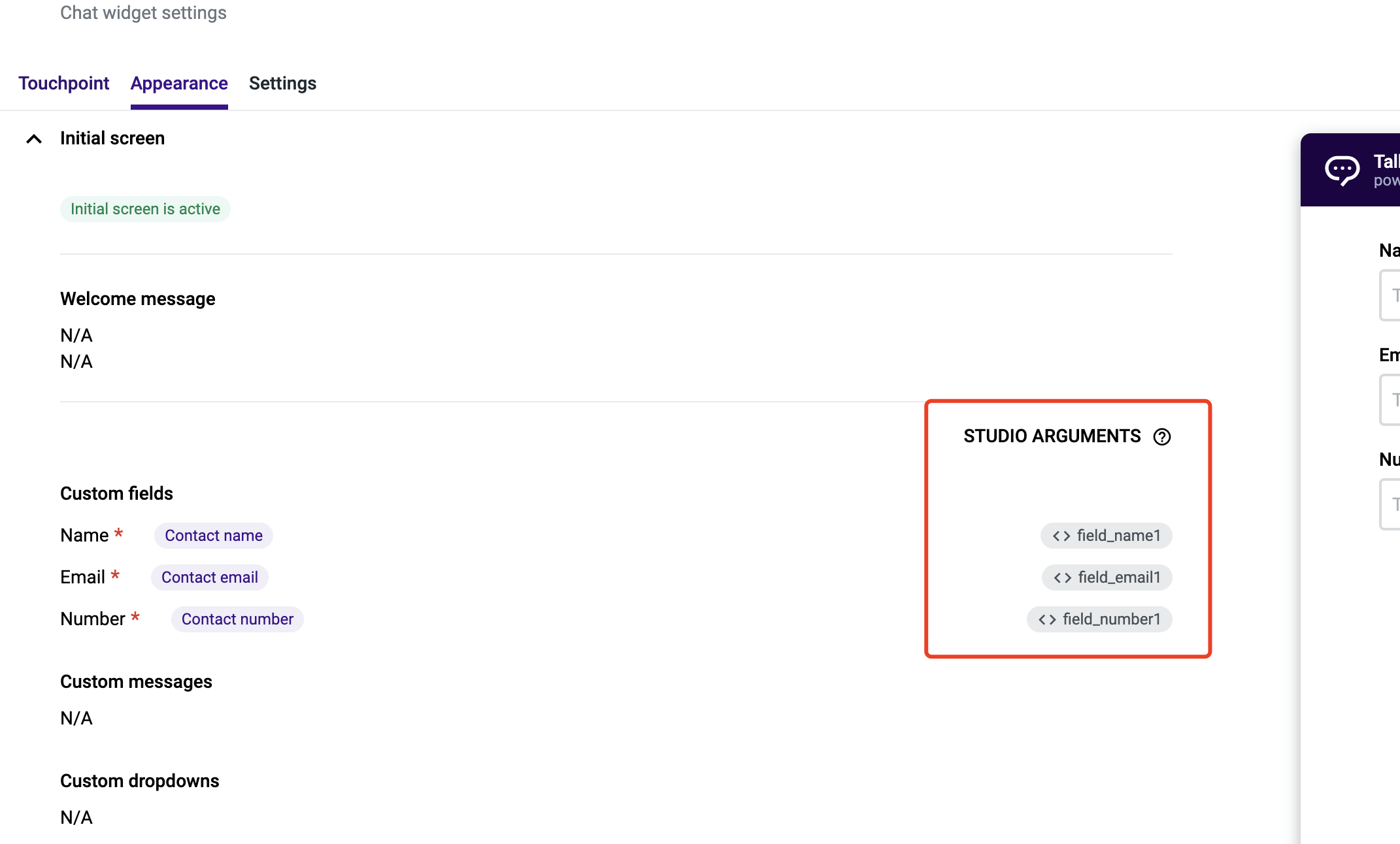
If default values are set for all fields, the chat will automatically skip the initial screen form stage and be automatically passed to the TalkDesk system when the conversation starts.
<!-- Start of Talkdesk Code -->
<script>
var webchat;
(function(window, document, node, props, configs) {
if (window.TalkdeskChatSDK) {
console.error("TalkdeskChatSDK already included");
return;
}
var divContainer = document.createElement("div");
divContainer.id = node;
document.body.appendChild(divContainer);
var src = "https://talkdeskchatsdk.talkdeskapp.com/talkdeskchatsdk.js";
var script = document.createElement("script");
var firstScriptTag = document.getElementsByTagName("script")[0];
script.type = "text/javascript";
script.charset = "UTF-8";
script.id = "tdwebchatscript";
script.src = src;
script.async = true;
firstScriptTag.parentNode.insertBefore(script, firstScriptTag);
script.onload = function() {
webchat = TalkdeskChatSDK(node, props);
webchat.init(configs);
+ // Note: Set the default value of the Initial screen form here. If default values are set for all fields, Talkdesk Chat will automatically skip the Initial screen stage and go directly to the conversation ui.
+ webchat.setCustomFieldDefaultValues({
+ "field_email1": "[email protected]",
+ "field_number1": "+1",
+ });
/*
* Send custom data from your website to TalkDesk!
* If you would like to do it, you need to remove the following commented code and
* modify the webchat.setContextParam parameters to pass in the data you need.
*/
/*function setContext() {
webchat.setContextParam({ "var1": "value1", "var2": "value2", "var3": 100 })
}
// Send data when the chat conversation is initiated
webchat.onConversationStart = function() {
setContext()
}
// Send data when the chat widget is open
webchat.onOpenWebchat = function() {
setContext()
}*/
};
})(
window,
document,
"tdWebchat",
{ touchpointId: "<customer touchpointId>", accountId: "", region: "<customer td region>" },
{ enableValidation: false, enableEmoji: true, enableUserInput: true, enableAttachments: true }
);
</script>
<!-- End of Talkdesk Code -->
Updated 4 months ago